Ethereum Data Retrieval with Binance Socket Manager using Python
As a beginner in cryptocurrency trading, understanding how to retrieve data from the Ethereum network can be a challenging task. In this article, we will walk you through the steps of setting up the BinanceSocketManager
library and retrieving Ethereum market data.
Prerequisites:
Before diving into the code, make sure you have:
- Python installed on your computer.
- The
binance
library installed (pip install binance-sdk
)
- A Binance API account with a valid access token
Installing the required libraries:
You can install the required libraries using pip:
pip install -U binance-sdk
Setting up the BinanceSocketManager:
The BinanceSocketManager
is used to establish a WebSocket connection to the Binance API. To use it, you need to create an instance of the Client
class and pass in your access token:
import asyncio
from binance import AsyncClient
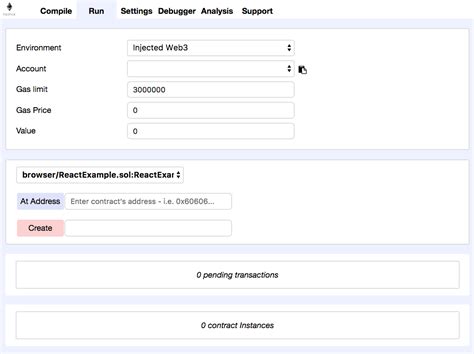
Replace with your Binance API access token
access_token = "YOUR_BINANCE_API_ACCESS_TOKEN"
async def retrieve_ethermarket_data():
Create a BinanceSocketManager instance
client = AsyncClient(access_token=access_token)
Define the WebSocket endpoint and callback function
async def on_message(data):
Process the incoming message (in this case, we'll just print it)
print(f"Received data from Binance: {data}")
Subscribe to the Ethereum market data channel
client.socketManager.subscribe(
symbol="ETH",
The Ethereum symbol (e.g., ETH/USDT)
callback=on_message
Callback function to handle incoming messages
)
async def main():
await retrieve_ethermarket_data()
Run the asyncio event loop
asyncio.run(main())
What’s happening in this code:
- We create an instance of
AsyncClient
using your Binance API access token.
- We define a callback function
on_message
that will be executed when new data is received from Binance.
- We subscribe to the Ethereum market data channel by passing in the symbol (
ETH
) and thecallback
function (in this case,on_message
).
- The
asyncio.run(main())
line runs the asyncio event loop.
What’s next?
This code sets up a basic WebSocket connection to Binance and subscribes to the Ethereum market data channel. To retrieve more detailed market data, such as order book, candlestick chart, or API call counts, you’ll need to modify the callback function accordingly. You can also explore other features like price charts, technical indicators, and more.
Tips and Variations:
- To handle errors, add try-except blocks around your code.
- Use a
while True
loop instead ofasyncio.run(main())
for continuous execution.
- Experiment with different callback functions to retrieve additional data or handle specific events.
- Consider using the
binance-sdk
library’s other features, like retrieving prices from exchanges or analyzing market trends.
Conclusion:
In this article, you learned how to use the BinanceSocketManager
library in Python to establish a WebSocket connection with Binance and retrieve Ethereum market data. With practice and exploration, you’ll be able to customize your code to suit your specific needs and gain valuable insights into the Ethereum ecosystem.